In this tutorial, we'll walk you through setting up the Stiddle Pixel for tracking with a headless Shopify Store that uses NextJS as the store frontend.
Step 1 - Get Stiddle Pixel
Navigate to settings screen, select the pixel tab, and click 'Copy Pixel Code' under 'Stiddle Pixel for Shopify'.
If the code is successfully copied to your clipboard, you should see a toaster on the top right of your screen like this:

Step 2 - Setup the Non-Checkout Pages
There are three ways to integrate the Stiddle Pixel with a NextJS store. We strongly suggest opting for method #1 as the primary choice.
Method #2 should only be considered if method #1 fails to function properly.
Method #3 should only be considered if method #1 and method #2 fails to function properly.
You only need to implement any of the three methods.
Method #1 (Recommended)
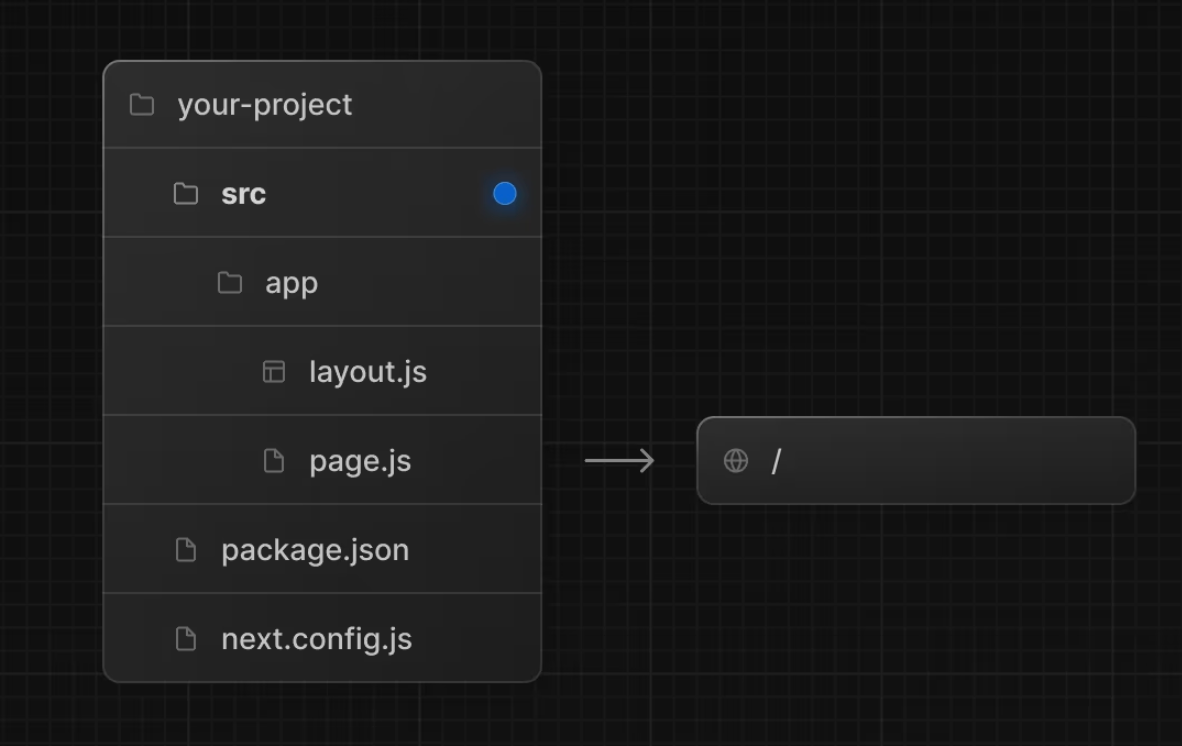
1. Locate your base layout file. Using the NextJS 14 recommended layout above - you can locate the layout.js file (or layout.tsx if you're using ts) within your frontend repository. This file should be found within src folder -> app folder -> layout.js
Alternatively, you can also locate a page like _app.tsx or _app.js within the pages folder that can be found with src folder -> pages folder -> _app.tsx

2. Import Head from 'next/head' and create a <Head><Head/> tag pair above component like the example below.
import '../styles/global.css';
import Head from 'next/head';
import type { AppProps } from 'next/app';
const MyApp = ({ Component, pageProps }: AppProps) => {
return (
<>
<Head>
</Head>
<Component {...pageProps} />
</>)
};
export default MyApp;
3. Then create a div tag and use dangerouslySetInnerHTML to create the following code snippet:
<div dangerouslySetInnerHTML={{__html: `PASTE YOUR PIXEL CODE HERE`}} />
Add the above pixel code from your clipboard to within the <Head></Head> tag, when you're done, it should look like below:
import '../styles/global.css';
import Head from 'next/head';
import type { AppProps } from 'next/app';
const MyApp = ({ Component, pageProps }: AppProps) => {
return (
<>
<Head>
<div
dangerouslySetInnerHTML={{__html: `<script type="text/javascript" defer src='//tracking.stiddlepixel.com/' id="stiddle-script" apiKey="YOUR API KEY"></script>`}}
/>
</Head>
<Component {...pageProps} />
</>)
};
export default MyApp;
Method #2 - Use public/static (Recommended Fallback)
1. Create a folder for your static files in :
<root>/public/static/script.js
2. Then, in the index.js at <root>/pages/index.js
, paste the script in between the <Head /> tags.
import Head from 'next/head';
import MyAwesomeComponent from '../components/mycomponent';
export default () => (
<div>
<Head>
<script type="text/javascript" defer src='//tracking.stiddlepixel.com/' id="stiddle-script" apiKey="YOUR API KEY"></script>
</Head>
<MyAwesomeComponent />
</div>
)
Method #3 - Modify Root Layout (Less Recommended Fallback)
Next.js uses a root layout component to wrap all pages. We'll modify this component to include the Stiddle Pixel script.
Step 1: Convert the Stiddle Pixel into a NextJS Script Component
Let's start with the original script tag you want to convert:
<script type="text/javascript" defer src="//tracking.stiddlepixel.com/" id="stiddle-script" apiKey="SOME API KEY"></script>
1. Identify the Attributes of the Script Tag
The original script tag has several attributes:
- type="text/javascript"
- defer
- src="//tracking.stiddlepixel.com/"
- id="stiddle-script"
- apiKey="SOME API KEY"
2. Install Next.js Script Component
Next.js provides a Script
component to manage script loading. First, ensure you have Next.js installed and set up.
3. Import the Script Component
In the file where you want to include the script (typically _app.js
or _app.tsx
), import the Script
component from next/script
.
Add import Script from 'next/script';
to the top of your file.
4. Use the Script Component
Replace the traditional script tag with the Script
component from Next.js.
- Replace src attribute. Use the src prop.
- Replace 'id' attribute. Use the id prop.
- Set 'strategy' prop. Use
strategy="lazyOnload"
to defer loading. - Embed the
apiKey
as a query parameter in thesrc
URL.
<Script
src="//tracking.stiddlepixel.com/?apiKey=YOUR API KEY"
id="stiddle-script"
strategy="lazyOnload"
/>
5. Add the Script tag we created before the closing body tag in _app.js
// pages/_app.js
import React from 'react';
import { ConfigProvider } from 'antd';
import Script from 'next/script';
import AntdRegistry from '../components/AntdRegistry'; // Adjust the import path as necessary
export default function MyApp({ Component, pageProps }) {
return (
<html lang="en">
<body>
<div>
<AntdRegistry>
<ConfigProvider>
<Component {...pageProps} />
</ConfigProvider>
</AntdRegistry>
</div>
<Script
src="//tracking.stiddlepixel.com/?apiKey=YOUR API KEY"
id="stiddle-script"
strategy="lazyOnload"
/>
</body>
</html>
);
}
Step 3 - Setup the checkout page
If you are using customer events, refer to this guide.
If you're currently utilizing checkout.liquid in conjunction with extra checkout script, it's recommended to shift to customer events with the provided instructions, as Shopify will be phasing out the extra checkout script by August 2024.
Step 4 - Test your store to confirm that Stiddle is tracking properly.
1. Run Your Next.js Application
Start your Next.js application using the following command: npm run dev
Open your browser and navigate to the URL where your application is running (e.g., http://localhost:3000 or your real domain if changes have been published
).
2. Open Developer Tools
Right-click anywhere on the page and select "Inspect" or press Ctrl+Shift+I for Windows and Linux or Cmd+Option+I for Mac to open the browser developer tools.

3. Go to the Network Tab
In the Developer Tools, click on the "Network" tab. This tab allows you to see all network requests made by the page.

4. Filter Network Requests
- In the "Network" tab, locate the "Filter" box.
- Type 'track' in the filter box to narrow down the list of network requests to only those that contain "track" in the URL. This helps you find the Stiddle Pixel's tracking calls.

5. Check the Network Request
- Look for a network request to 'api.stiddle.com/api/v1/contacts/track'. The filtered results should help you identify this request quickly.
- Click on this request to view its details.
6. Verify the Response
- In the details pane for the network request, check the "Status" column.
- Ensure the status code is '201 Created', indicating a successful request.

7. Inspect Response Details
- After clicking on the network request, a new pane will show detailed information about the request.
- Optionally, check the profiles section of the platform to verify that your request has been saved correctly in our database.
Conclusion
And that's it. By following these steps, you can ensure the Stiddle Pixel script is properly implemented and successfully making tracking calls in a NextJS headless Shopify store setup.
For more information or assistance, contact Stiddle support.